Note
This page was generated from circuit-examples//D.Qubit-couplers//32-TwoTransmonsDirectCoupling.ipynb.
Direct Coupler (transmon-transmon)#
[1]:
%load_ext autoreload
%autoreload 2
import qiskit_metal as metal
from qiskit_metal import designs, draw
from qiskit_metal import MetalGUI, Dict, Headings
import pyEPR as epr
Create the design in Metal#
Setup a design of a given dimension. Dimensions will be respected in the design rendering. Note that the design size extends from the origin into the first quadrant.
[2]:
design = designs.DesignPlanar({}, True)
design.chips.main.size['size_x'] = '2mm'
design.chips.main.size['size_y'] = '2mm'
gui = MetalGUI(design)
Create two transmons with one readout resonator and move it to the center of the chip previously defined.
[3]:
from qiskit_metal.qlibrary.qubits.transmon_pocket import TransmonPocket
from qiskit_metal.qlibrary.tlines.straight_path import RouteStraight
# Just FYI, RoutePathFinder is another QComponent that could have been used instead of RouteStraight.
[4]:
# Be aware of the options for TransmonPocket
TransmonPocket.get_template_options(design)
[4]:
{'pos_x': '0um',
'pos_y': '0um',
'connection_pads': {},
'_default_connection_pads': {'pad_gap': '15um',
'pad_width': '125um',
'pad_height': '30um',
'pad_cpw_shift': '5um',
'pad_cpw_extent': '25um',
'cpw_width': 'cpw_width',
'cpw_gap': 'cpw_gap',
'cpw_extend': '100um',
'pocket_extent': '5um',
'pocket_rise': '65um',
'loc_W': '+1',
'loc_H': '+1'},
'chip': 'main',
'pad_gap': '30um',
'inductor_width': '20um',
'pad_width': '455um',
'pad_height': '90um',
'pocket_width': '650um',
'pocket_height': '650um',
'orientation': '0',
'hfss_wire_bonds': False,
'q3d_wire_bonds': False,
'hfss_inductance': '10nH',
'hfss_capacitance': 0,
'hfss_resistance': 0,
'hfss_mesh_kw_jj': 7e-06,
'q3d_inductance': '10nH',
'q3d_capacitance': 0,
'q3d_resistance': 0,
'q3d_mesh_kw_jj': 7e-06,
'gds_cell_name': 'my_other_junction'}
[5]:
RouteStraight.get_template_options(design)
[5]:
{'pin_inputs': {'start_pin': {'component': '', 'pin': ''},
'end_pin': {'component': '', 'pin': ''}},
'fillet': '0',
'lead': {'start_straight': '0mm',
'end_straight': '0mm',
'start_jogged_extension': '',
'end_jogged_extension': ''},
'total_length': '7mm',
'chip': 'main',
'layer': '1',
'trace_width': 'cpw_width',
'hfss_wire_bonds': False,
'q3d_wire_bonds': False}
[6]:
q1 = TransmonPocket(design, 'Q1', options = dict(
pad_width = '425 um',
pocket_height = '650um',
connection_pads=dict(
readout = dict(loc_W=+1,loc_H=+1, pad_width='200um')
)))
q2 = TransmonPocket(design, 'Q2', options = dict(
pos_x = '1.0 mm',
pad_width = '425 um',
pocket_height = '650um',
connection_pads=dict(
readout = dict(loc_W=-1,loc_H=+1, pad_width='200um')
)))
bus = RouteStraight(design, 'coupler', Dict(
pin_inputs=Dict(
start_pin=Dict(component='Q1', pin='readout'),
end_pin=Dict(component='Q2', pin='readout')), ))
gui.rebuild()
gui.autoscale()
[7]:
# Get a list of all the qcomponents in QDesign and then zoom on them.
all_component_names = design.components.keys()
gui.zoom_on_components(all_component_names)
[8]:
#Save screenshot as a .png formatted file.
gui.screenshot()
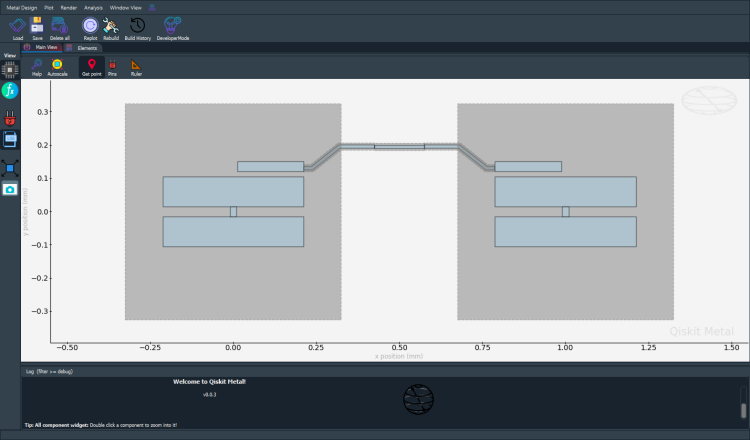
[9]:
# Screenshot the canvas only as a .png formatted file.
gui.figure.savefig('shot.png')
from IPython.display import Image, display
_disp_ops = dict(width=500)
display(Image('shot.png', **_disp_ops))
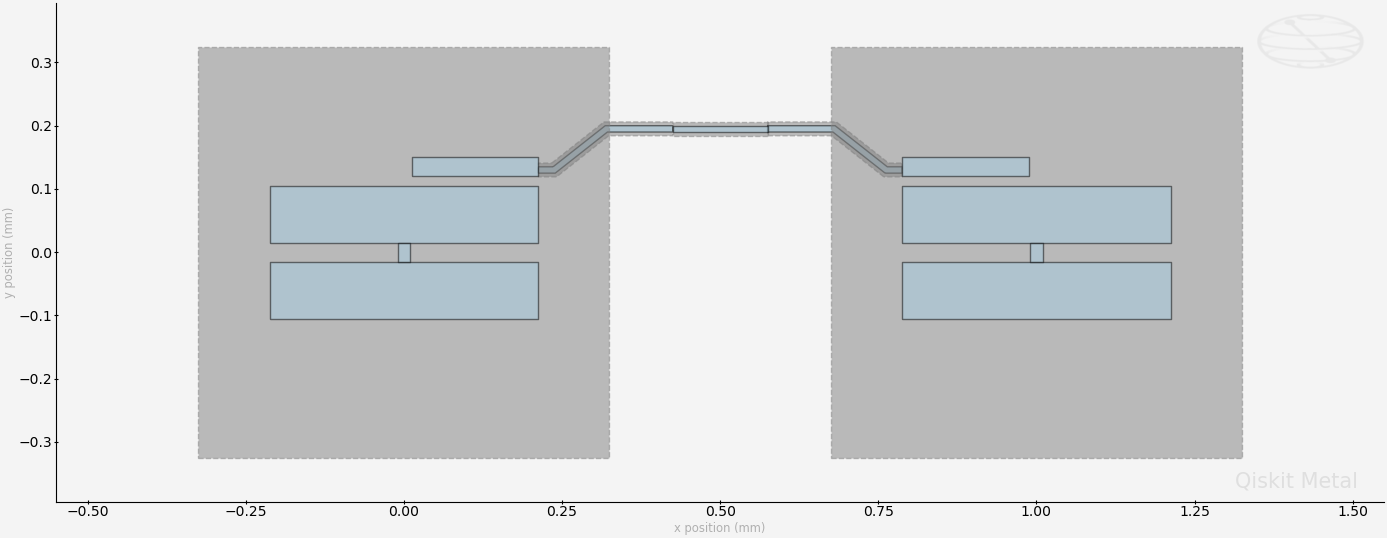
[10]:
# Closing the Qiskit Metal GUI
gui.main_window.close()
[10]:
True
[ ]:
For more information, review the Introduction to Quantum Computing and Quantum Hardware lectures below
|
Lecture Video | Lecture Notes | Lab |
|
Lecture Video | Lecture Notes | Lab |
|
Lecture Video | Lecture Notes | Lab |
|
Lecture Video | Lecture Notes | Lab |
|
Lecture Video | Lecture Notes | Lab |
|
Lecture Video | Lecture Notes | Lab |