Note
This page was generated from docs/tutorials/01_algorithms_introduction.ipynb.
An Introduction to Algorithms using Qiskit#
This introduction to algorithms using Qiskit provides a high-level overview to get started with the qiskit_algorithms
library.
How is the algorithm library structured?#
qiskit_algorithms
provides a number of algorithms grouped by category, according to the task they can perform. For instance Minimum Eigensolvers
to find the smallest eigen value of an operator, for example ground state energy of a chemistry Hamiltonian or a solution to an optimization problem when expressed as an Ising Hamiltonian. There are Time Evolvers
for the time evolution of quantum systems and
Amplitude Estimators
for value estimation that can be used say in financial applications. The full set of categories can be seen in the documentation link above.
Algorithms are configurable, and part of the configuration will often be in the form of smaller building blocks. For instance VQE
, the Variational Quantum Eigensolver, it takes a trial wavefunction, in the form of a QuantumCircuit
and a classical optimizer among other things.
Let’s take a look at an example to construct a VQE instance. Here, TwoLocal
is the variational form (trial wavefunction), a parameterized circuit which can be varied, and SLSQP
a classical optimizer. These are created as separate instances and passed to VQE when it is constructed. Trying, for example, a different classical optimizer, or variational form is simply a case of creating an instance of the one you want and passing it into VQE.
[1]:
from qiskit_algorithms.optimizers import SLSQP
from qiskit.circuit.library import TwoLocal
num_qubits = 2
ansatz = TwoLocal(num_qubits, "ry", "cz")
optimizer = SLSQP(maxiter=1000)
Let’s draw the ansatz so we can see it’s a QuantumCircuit
where θ[0] through θ[7] will be the parameters that are varied as VQE optimizer finds the minimum eigenvalue. We’ll come back to the parameters later in a working example below.
[2]:
ansatz.decompose().draw("mpl")
[2]:
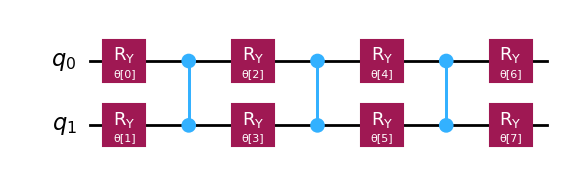
But more is needed before we can run the algorithm so let’s get to that next.
How to run an algorithm?#
Algorithms rely on the primitives to evaluate expectation values or sample circuits. The primitives can be based on a simulator or real device and can be used interchangeably in the algorithms, as they all implement the same interface.
In the VQE, we have to evaluate expectation values, so for example we can use the qiskit.primitives.Estimator which is shipped with the default Qiskit installation.
[3]:
from qiskit.primitives import Estimator
estimator = Estimator()
This estimator uses an exact, statevector simulation to evaluate the expectation values. We can also use a shot-based and noisy simulators or real backends instead. For more information of the simulators you can check out Qiskit Aer and for the actual hardware Qiskit IBM Runtime.
With all the ingredients ready, we can now instantiate the VQE:
[4]:
from qiskit_algorithms import VQE
vqe = VQE(estimator, ansatz, optimizer)
Now we can call the compute_mininum_eigenvalue() method. The latter is the interface of choice for the application modules, such as Nature and Optimization, in order that they can work interchangeably with any algorithm within the specific category.
A complete working example#
Let’s put what we have learned from above together and create a complete working example. VQE will find the minimum eigenvalue, i.e. minimum energy value of a Hamiltonian operator and hence we need such an operator for VQE to work with. Such an operator is given below. This was originally created by the Nature application module as the Hamiltonian for an H2 molecule at 0.735A interatomic distance. It’s a sum of Pauli terms as below.
[5]:
from qiskit.quantum_info import SparsePauliOp
H2_op = SparsePauliOp.from_list(
[
("II", -1.052373245772859),
("IZ", 0.39793742484318045),
("ZI", -0.39793742484318045),
("ZZ", -0.01128010425623538),
("XX", 0.18093119978423156),
]
)
So let’s run VQE and print the result object it returns.
[6]:
result = vqe.compute_minimum_eigenvalue(H2_op)
print(result)
{ 'aux_operators_evaluated': None,
'cost_function_evals': 63,
'eigenvalue': -1.857274987488336,
'optimal_circuit': <qiskit.circuit.library.n_local.two_local.TwoLocal object at 0x7f40547b01c0>,
'optimal_parameters': { ParameterVectorElement(θ[0]): 3.8945460081931684,
ParameterVectorElement(θ[1]): -4.038741430885725,
ParameterVectorElement(θ[2]): -3.8643049669872602,
ParameterVectorElement(θ[3]): 5.988742233569182,
ParameterVectorElement(θ[4]): -2.4148614664173094,
ParameterVectorElement(θ[5]): 2.603313461817561,
ParameterVectorElement(θ[6]): -2.015152982019603,
ParameterVectorElement(θ[7]): 0.18306366875278743},
'optimal_point': array([ 3.89454601, -4.03874143, -3.86430497, 5.98874223, -2.41486147,
2.60331346, -2.01515298, 0.18306367]),
'optimal_value': -1.857274987488336,
'optimizer_evals': None,
'optimizer_result': <qiskit_algorithms.optimizers.optimizer.OptimizerResult object at 0x7f40093ef370>,
'optimizer_time': 0.1451117992401123}
From the above result we can see the number of cost function (=energy) evaluations the optimizer took until it found the minimum eigenvalue of \(\approx -1.85727\) which is the electronic ground state energy of the given H2 molecule. The optimal parameters of the ansatz can also be seen which are the values that were in the ansatz at the minimum value.
Updating the primitive inside VQE#
To close off let’s also change the estimator primitive inside the a VQE. Maybe you’re satisfied with the simulation results and now want to use a shot-based simulator, or run on hardware!
In this example we’re changing to a shot-based estimator, still using Qiskit’s reference primitive. However, you could replace the primitive by e.g. Qiskit Aer’s estimator (qiskit_aer.primitives.Estimator) or even a real backend (qiskit_ibm_runtime.Estimator).
For noisy loss functions, the SPSA optimizer typically performs well, so we also update the optimizer. See also the noisy VQE tutorial for more details on shot-based and noisy simulations.
[7]:
from qiskit_algorithms.optimizers import SPSA
estimator = Estimator(options={"shots": 1000})
vqe.estimator = estimator
vqe.optimizer = SPSA(maxiter=100)
result = vqe.compute_minimum_eigenvalue(operator=H2_op)
print(result)
{ 'aux_operators_evaluated': None,
'cost_function_evals': 200,
'eigenvalue': -1.8571130246482759,
'optimal_circuit': <qiskit.circuit.library.n_local.two_local.TwoLocal object at 0x7f40093b9400>,
'optimal_parameters': { ParameterVectorElement(θ[0]): 1.4792895495791105,
ParameterVectorElement(θ[1]): 1.9126080137582482,
ParameterVectorElement(θ[2]): 5.836191381022055,
ParameterVectorElement(θ[3]): -1.7709566376879826,
ParameterVectorElement(θ[4]): 2.9809753809592094,
ParameterVectorElement(θ[5]): 7.159705091586209,
ParameterVectorElement(θ[6]): -4.556668755826434,
ParameterVectorElement(θ[7]): -5.282330615031699},
'optimal_point': array([ 1.47928955, 1.91260801, 5.83619138, -1.77095664, 2.98097538,
7.15970509, -4.55666876, -5.28233062]),
'optimal_value': -1.8571130246482759,
'optimizer_evals': None,
'optimizer_result': <qiskit_algorithms.optimizers.optimizer.OptimizerResult object at 0x7f400c525670>,
'optimizer_time': 0.6076390743255615}
Note: We do not fix the random seed in the simulators here, so re-running gives slightly varying results.
This concludes this introduction to algorithms using Qiskit. Please check out the other algorithm tutorials in this series for both broader as well as more in depth coverage of the algorithms.
[8]:
import tutorial_magics
%qiskit_version_table
%qiskit_copyright
Version Information
Software | Version |
---|---|
qiskit | 1.0.2 |
qiskit_algorithms | 0.3.0 |
System information | |
Python version | 3.8.18 |
OS | Linux |
Wed Apr 10 17:18:24 2024 UTC |
This code is a part of a Qiskit project
© Copyright IBM 2017, 2024.
This code is licensed under the Apache License, Version 2.0. You may
obtain a copy of this license in the LICENSE.txt file in the root directory
of this source tree or at http://www.apache.org/licenses/LICENSE-2.0.
Any modifications or derivative works of this code must retain this
copyright notice, and modified files need to carry a notice indicating
that they have been altered from the originals.