Tφ Characterization¶
We therefore create a composite experiment consisting of a
From the
Note
This tutorial requires the qiskit-aer and qiskit-ibm-runtime
packages to run simulations. You can install them with python -m pip
install qiskit-aer qiskit-ibm-runtime
.
import numpy as np
import qiskit
from qiskit_experiments.library.characterization import Tphi
# An Aer simulator
from qiskit_aer import AerSimulator
from qiskit_aer.noise import NoiseModel
from qiskit_ibm_runtime.fake_provider import FakePerth
# Create a pure relaxation noise model for AerSimulator
noise_model = NoiseModel.from_backend(
FakePerth(), thermal_relaxation=True, gate_error=False, readout_error=False
)
# Create a fake backend simulator
backend = AerSimulator.from_backend(FakePerth(), noise_model=noise_model)
# Time intervals to wait before measurement for t1 and t2
delays_t1 = np.arange(1e-6, 300e-6, 10e-6)
delays_t2 = np.arange(1e-6, 50e-6, 2e-6)
By default, the Tphi
experiment will use the Hahn echo experiment for its transverse
relaxation time estimate. We can see that the component experiments of the batch
Tphi
experiment are what we expect for T1
and T2Hahn
:
exp = Tphi(physical_qubits=(0,), delays_t1=delays_t1, delays_t2=delays_t2, num_echoes=1)
exp.component_experiment(0).circuits()[-1].draw(output="mpl", style="iqp")

exp.component_experiment(1).circuits()[-1].draw(output="mpl", style="iqp")
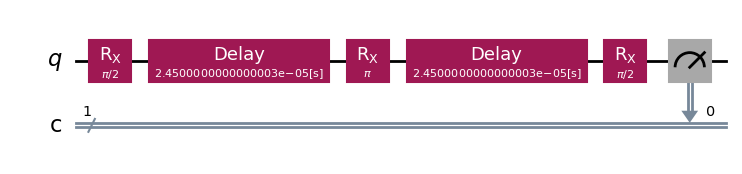
Run the experiment and print results:
expdata = exp.run(backend=backend, seed_simulator=100).block_for_results()
display(expdata.analysis_results("T_phi", dataframe=True))
name | experiment | components | value | quality | backend | run_time | unit | chisq | |
---|---|---|---|---|---|---|---|---|---|
6eb15733 | T_phi | Tphi | [Q0] | -0.0004+/-0.0008 | bad | aer_simulator_from(fake_perth) | None | s | None |
You can also retrieve the results and figures of the constituent experiments. T1
:
display(expdata.analysis_results("T1", dataframe=True))
display(expdata.figure(0))
name | experiment | components | value | quality | backend | run_time | unit | chisq | |
---|---|---|---|---|---|---|---|---|---|
dd7bcc1d | T1 | T1 | [Q0] | (5.59+/-0.08)e-05 | good | aer_simulator_from(fake_perth) | None | s | 1.247985 |
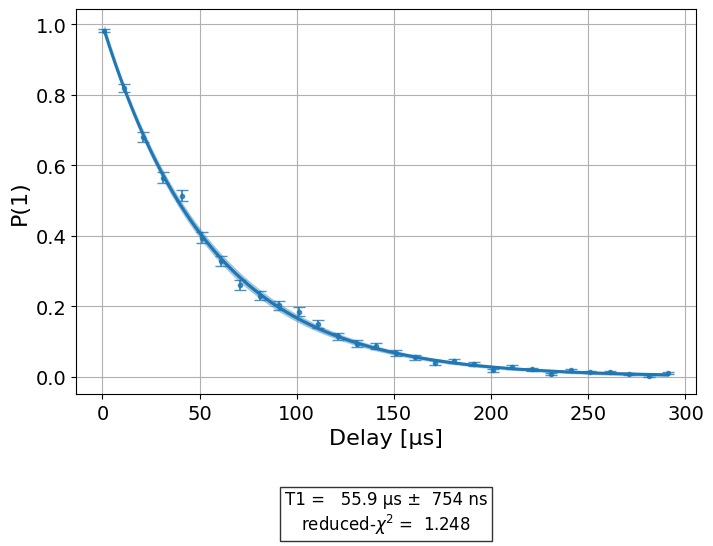
And T2Hahn
:
display(expdata.analysis_results("T2", dataframe=True))
display(expdata.figure(1))
name | experiment | components | value | quality | backend | run_time | unit | chisq | |
---|---|---|---|---|---|---|---|---|---|
819de62b | T2 | T2Hahn | [Q0] | 0.00015+/-0.00011 | bad | aer_simulator_from(fake_perth) | None | s | 1.502093 |
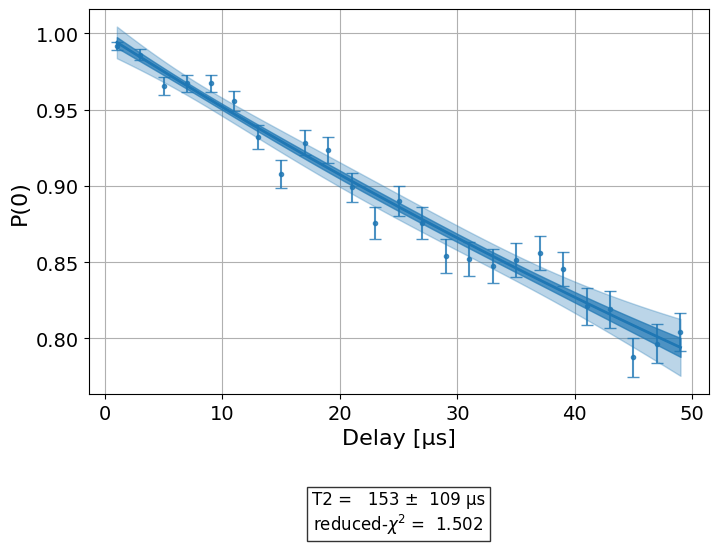
Let’s now run the experiment with T2Ramsey
by setting the t2type
option to
ramsey
and specifying osc_freq
. Now the second component experiment is a Ramsey
experiment:
exp = Tphi(physical_qubits=(0,),
delays_t1=delays_t1,
delays_t2=delays_t2,
t2type="ramsey",
osc_freq=1e5)
exp.component_experiment(1).circuits()[-1].draw(output="mpl", style="iqp")
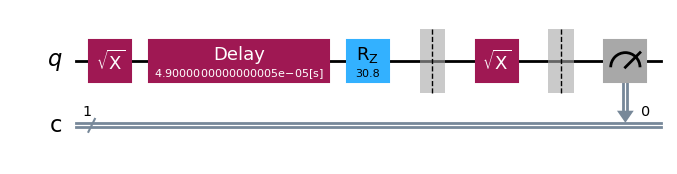
Run and display results:
expdata = exp.run(backend=backend, seed_simulator=100).block_for_results()
display(expdata.analysis_results("T_phi", dataframe=True))
display(expdata.figure(1))
name | experiment | components | value | quality | backend | run_time | unit | chisq | |
---|---|---|---|---|---|---|---|---|---|
1d5c63cc | T_phi | Tphi | [Q0] | 0.00053+/-0.00017 | good | aer_simulator_from(fake_perth) | None | s | None |
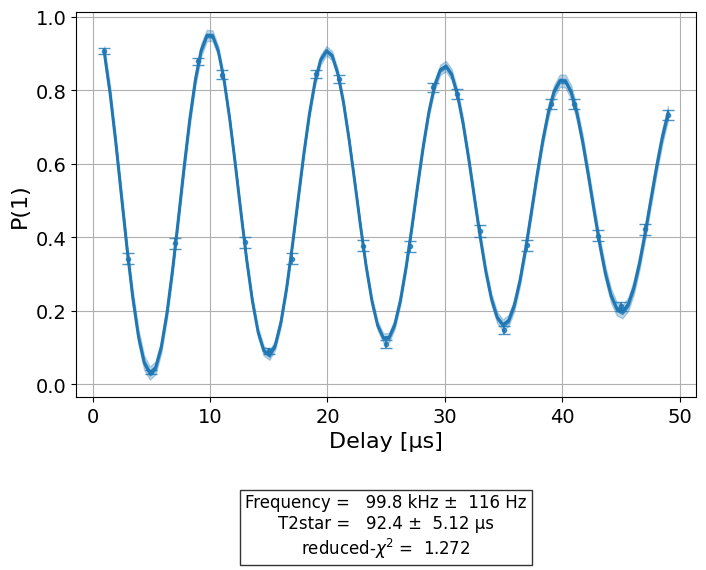
Because we are using a simulator that doesn’t model inhomogeneous broadening, the
References¶
See also¶
API documentation:
Tphi