T2* Ramsey Characterization¶
The purpose of the T2Hahn
,
Since the detuning frequency is relatively small, we add a phase gate to the circuit to
enable better measurement. The actual frequency measured is the sum of the detuning
frequency and the user induced oscillation frequency (osc_freq
parameter).
import numpy as np
import qiskit
from qiskit_experiments.library import T2Ramsey
The circuits used for the experiment comprise the following steps:
Hadamard gate
Delay
RZ gate that rotates the qubit in the x-y plane
Hadamard gate
Measurement
The user provides as input a series of delays (in seconds) and the
oscillation frequency (in Hz). During the delay, we expect the qubit to
precess about the z-axis. If the p gate and the precession offset each
other perfectly, then the qubit will arrive at the
qubit = 0
# set the desired delays
delays = list(np.arange(1e-6, 50e-6, 2e-6))
# Create a T2Ramsey experiment. Print the first circuit as an example
exp1 = T2Ramsey((qubit,), delays, osc_freq=1e5)
print(exp1.circuits()[0])
┌────┐┌─────────────────┐┌─────────┐ ░ ┌────┐ ░ ┌─┐
q: ┤ √X ├┤ Delay(1e-06[s]) ├┤ Rz(π/5) ├─░─┤ √X ├─░─┤M├
└────┘└─────────────────┘└─────────┘ ░ └────┘ ░ └╥┘
c: 1/═════════════════════════════════════════════════╩═
0
We run the experiment on a simulated backend using Qiskit Aer with a pure T1/T2 relaxation noise model.
Note
This tutorial requires the qiskit-aer and qiskit-ibm-runtime
packages to run simulations. You can install them with python -m pip
install qiskit-aer qiskit-ibm-runtime
.
# A T1 simulator
from qiskit_ibm_runtime.fake_provider import FakePerth
from qiskit_aer import AerSimulator
from qiskit_aer.noise import NoiseModel
# Create a pure relaxation noise model for AerSimulator
noise_model = NoiseModel.from_backend(
FakePerth(), thermal_relaxation=True, gate_error=False, readout_error=False
)
# Create a fake backend simulator
backend = AerSimulator.from_backend(FakePerth(), noise_model=noise_model)
The resulting graph will have the form:
# Set scheduling method so circuit is scheduled for delay noise simulation
exp1.set_transpile_options(scheduling_method='asap')
# Run experiment
expdata1 = exp1.run(backend=backend, shots=2000, seed_simulator=101)
expdata1.block_for_results() # Wait for job/analysis to finish.
# Display the figure
display(expdata1.figure(0))
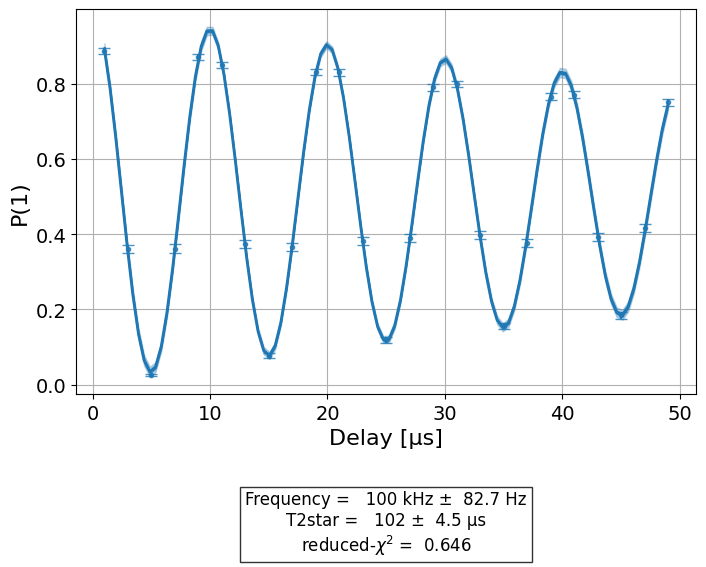
# Print results
for result in expdata1.analysis_results():
print(result)
AnalysisResult
- name: @Parameters_T2RamseyAnalysis
- value: CurveFitResult:
- fitting method: least_squares
- number of sub-models: 1
* F_cos_decay(x) = amp * exp(-x / tau) * cos(2 * pi * freq * x + phi) + base
- success: True
- number of function evals: 55
- degree of freedom: 20
- chi-square: 12.920802539385589
- reduced chi-square: 0.6460401269692795
- Akaike info crit.: -6.500930309783772
- Bayesian info crit.: -0.406551185442769
- init params:
* amp = 0.5
* tau = 0.00011350694107279987
* freq = 120071.20598218754
* phi = -1.5707963267948966
* base = 0.4761619190404797
- fit params:
* amp = 0.4909535980577468 ± 0.004917675368751884
* tau = 0.00010246327619765823 ± 4.5024424121587285e-06
* freq = 99952.75284201081 ± 82.7276550564961
* phi = 0.003658666993158401 ± 0.012651839512890825
* base = 0.5009230629507393 ± 0.0018222013166011644
- correlations:
* (freq, phi) = -0.8234936909699664
* (amp, tau) = -0.8140065430399878
* (freq, base) = -0.1713510143156256
* (amp, freq) = -0.11491601026425052
* (tau, phi) = -0.11201742411658946
* (amp, base) = -0.019330623607693696
* (tau, base) = 0.03640770514308672
* (tau, freq) = 0.06109075429281112
* (amp, phi) = 0.16116721298707007
* (phi, base) = 0.1637380874598672
- quality: good
- extra: <3 items>
- device_components: ['Q0']
- verified: False
AnalysisResult
- name: Frequency
- value: (9.995+/-0.008)e+04
- χ²: 0.6460401269692795
- quality: good
- extra: <3 items>
- device_components: ['Q0']
- verified: False
AnalysisResult
- name: T2star
- value: 0.000102+/-0.000005
- χ²: 0.6460401269692795
- quality: good
- extra: <3 items>
- device_components: ['Q0']
- verified: False
Providing initial user estimates¶
The user can provide initial estimates for the parameters to help the
analysis process. Because the curve is expected to decay toward
t2ramsey
and f
are the parameters of interest. Good estimates for them are values
computed in previous experiments on this qubit or a similar values
computed for other qubits.
user_p0={
"A": 0.5,
"T2star": 20e-6,
"f": 110000,
"phi": 0,
"B": 0.5
}
exp_with_p0 = T2Ramsey((qubit,), delays, osc_freq=1e5)
exp_with_p0.analysis.set_options(p0=user_p0)
exp_with_p0.set_transpile_options(scheduling_method='asap')
expdata_with_p0 = exp_with_p0.run(backend=backend, shots=2000, seed_simulator=101)
expdata_with_p0.block_for_results()
# Display fit figure
display(expdata_with_p0.figure(0))
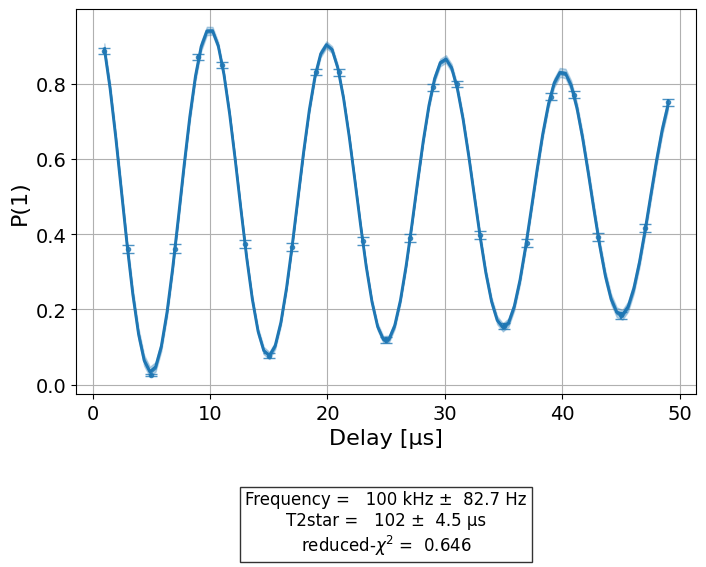
# Print results
for result in expdata_with_p0.analysis_results():
print(result)
AnalysisResult
- name: @Parameters_T2RamseyAnalysis
- value: CurveFitResult:
- fitting method: least_squares
- number of sub-models: 1
* F_cos_decay(x) = amp * exp(-x / tau) * cos(2 * pi * freq * x + phi) + base
- success: True
- number of function evals: 30
- degree of freedom: 20
- chi-square: 12.920802539387553
- reduced chi-square: 0.6460401269693776
- Akaike info crit.: -6.500930309779967
- Bayesian info crit.: -0.40655118543896407
- init params:
* amp = 0.5
* tau = 0.00011350694107279987
* freq = 100088.96797153035
* phi = 0.0
* base = 0.4761619190404797
- fit params:
* amp = 0.49095359392905114 ± 0.004917675333125186
* tau = 0.00010246328072257995 ± 4.502442770448915e-06
* freq = 99952.75286512234 ± 82.72765553370662
* phi = 0.0036586633504925546 ± 0.012651839431507947
* base = 0.5009230629493264 ± 0.0018222013158092263
- correlations:
* (freq, phi) = -0.8234936908975428
* (amp, tau) = -0.814006541969214
* (freq, base) = -0.1713510116625593
* (amp, freq) = -0.11491600400581126
* (tau, phi) = -0.11201741490831482
* (amp, base) = -0.019330626803089217
* (tau, base) = 0.036407708240404874
* (tau, freq) = 0.06109074531017117
* (amp, phi) = 0.16116720649724417
* (phi, base) = 0.16373808501693632
- quality: good
- extra: <3 items>
- device_components: ['Q0']
- verified: False
AnalysisResult
- name: Frequency
- value: (9.995+/-0.008)e+04
- χ²: 0.6460401269693776
- quality: good
- extra: <3 items>
- device_components: ['Q0']
- verified: False
AnalysisResult
- name: T2star
- value: 0.000102+/-0.000005
- χ²: 0.6460401269693776
- quality: good
- extra: <3 items>
- device_components: ['Q0']
- verified: False
See also¶
API documentation:
T2Ramsey